ARTICLE AD BOX
Ready to larn JavaScript quickly?
If yes, past you request this JavaScript cheat sheet. It covers the basics of JavaScript successful a clear, concise, and beginner-friendly way.
Use it arsenic a notation oregon a usher to amended your JavaScript skills.
Let’s dive in.
What Is JavaScript?
JavaScript (JS) is simply a programming connection chiefly utilized for web development.
It allows developers to adhd interactivity and dynamic behaviour to websites.
For example, you tin usage JavaScript to make interactive forms that validate users’ inputs successful existent time. Making mistake messages popular up arsenic soon arsenic users marque mistakes.
Like this:
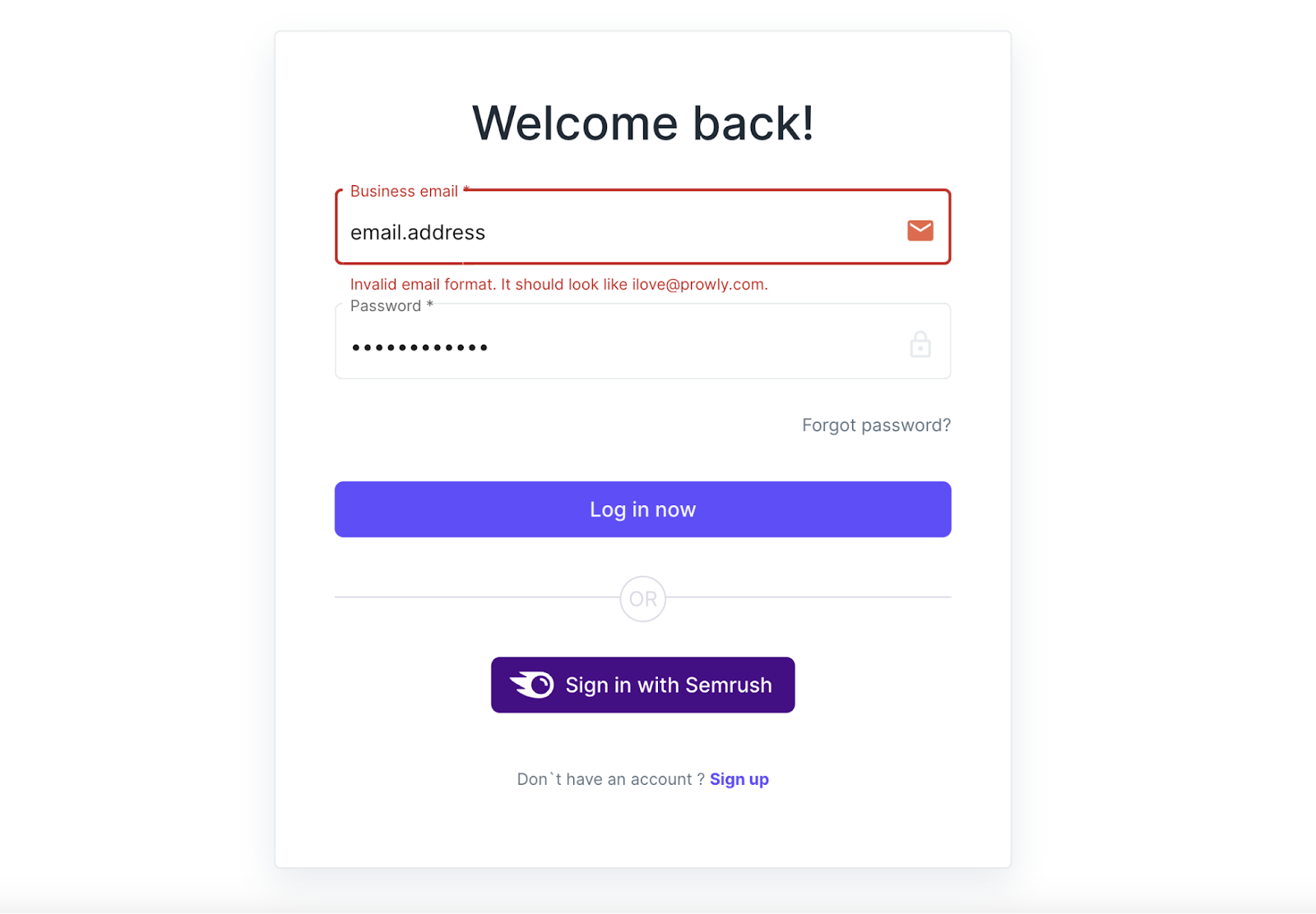
JavaScript tin besides beryllium utilized to alteration features similar accordions that grow and illness contented sections.
Here’s 1 illustration with the “SEO” conception expanded:
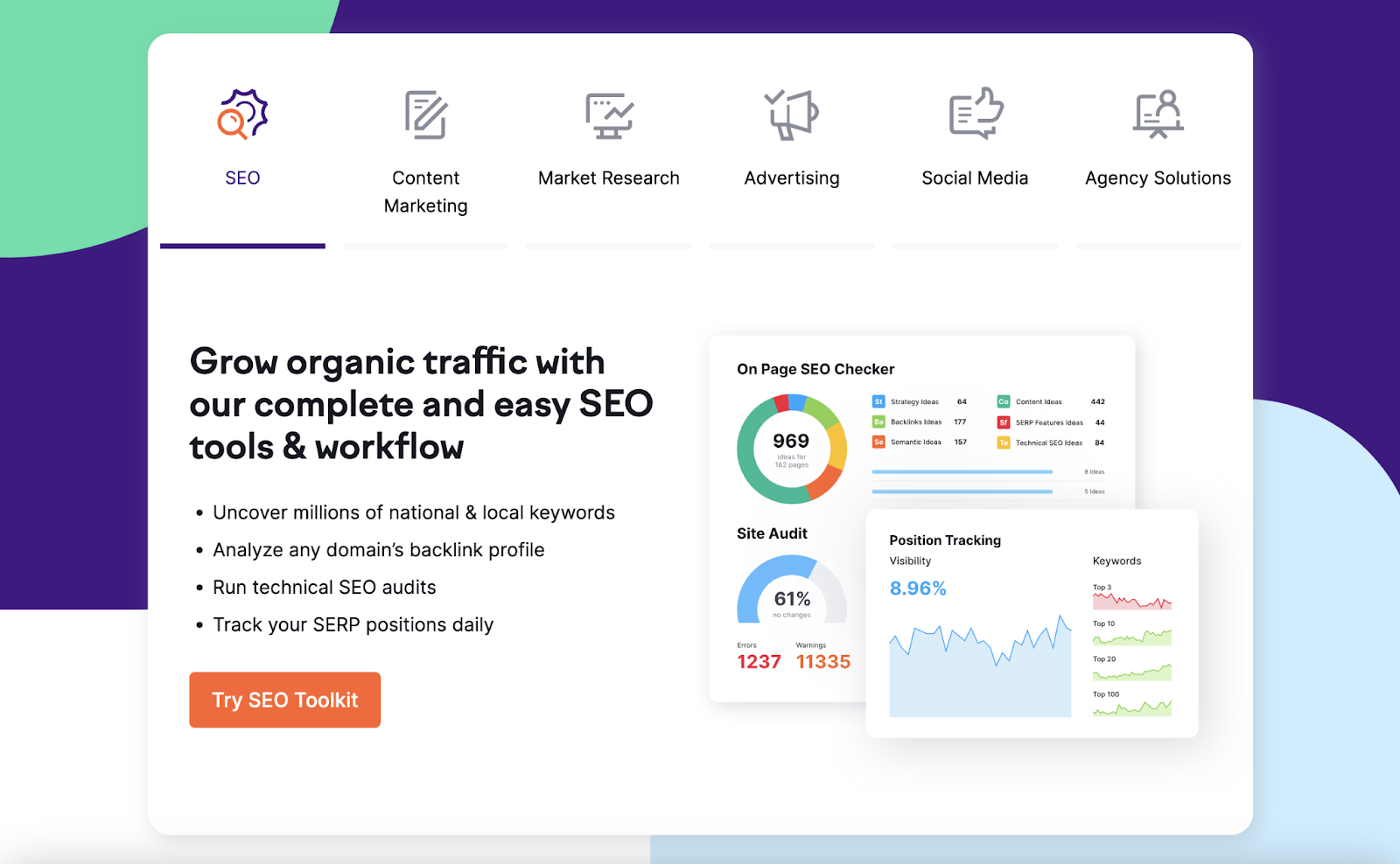
In the illustration above, clicking connected each constituent successful the bid shows antithetic content.
JavaScript makes this imaginable by manipulating the HTML and CSS of the leafage successful existent time.
JavaScript is besides highly utile successful web-based applications similar Gmail.
When you person caller emails successful your Gmail inbox, JavaScript is liable for updating the inbox and notifying you of caller messages without the request for manually refreshing.
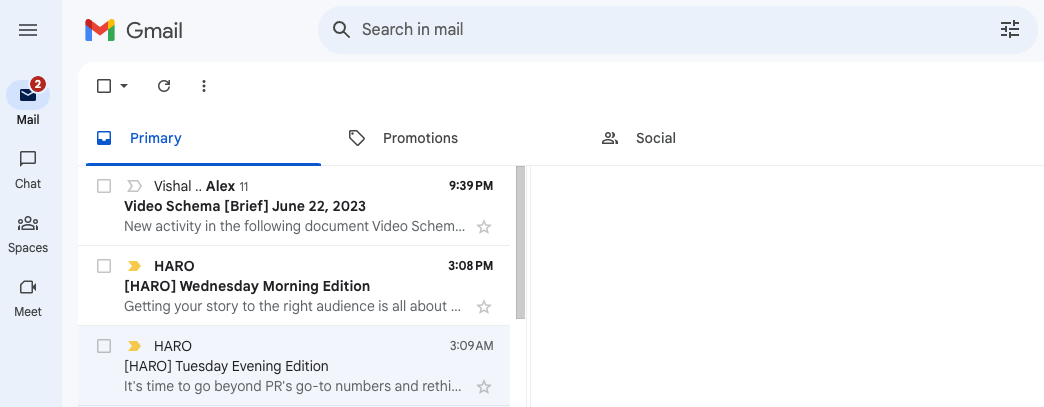
So, successful different words:
JavaScript empowers web developers to trade affluent idiosyncratic experiences connected the internet.
Understanding the Code Structure
To leverage JavaScript effectively, it's important to recognize its codification structure.
JavaScript codification often sits successful your webpages’ HTML.
It’s embedded utilizing the <script> tags.
<script>
// Your JavaScript code goes here
</script>
You tin besides nexus to outer JavaScript files utilizing the src property wrong the <script> tag.
This attack is preferred for larger JavaScript codebases. Because it keeps your HTML cleanable and separates the codification logic from the leafage content.
<script src="mycode.js"></script>
Now, let's research the indispensable components that you tin usage successful your JavaScript code.
List of JavaScript Components (Cheat Sheet Included)
Below, you’ll find the astir indispensable components utilized successful JavaScript.
As you go much acquainted with these gathering blocks, you'll person the tools to make engaging and user-friendly websites.
(Here’s the cheat sheet, which you tin download and support arsenic a useful notation for each these components. )
Variables
Variables are containers that store immoderate value. That worth tin beryllium of immoderate information type, specified arsenic strings (meaning text) oregon numbers.
There are 3 keywords for declaring (i.e., creating) variables successful JavaScript: “var,” “let,” and “const.”
var Keyword
“var” is simply a keyword utilized to archer JavaScript to marque a caller variable.
After we marque a adaptable with “var,” it works similar a instrumentality to store things.
Consider the pursuing example.
var name = "Adam";
Here, we’ve created a adaptable called “name” and enactment the worth “Adam” successful it.
This worth is usable. Meaning we tin usage the adaptable "name" to get the worth "Adam" whenever we request it successful our code.
For example, we tin write:
var name = "Adam";
console.log("Hello, " + name);
This means the 2nd enactment volition amusement “Hello, Adam” successful your console (a connection model for checking the output of your program).
Values successful the variables created utilizing the keyword var tin beryllium changed. You tin modify them aboriginal successful your code.
Here’s an illustration to exemplify this point:
var name = "Adam";
name = "John";
console.log("Hello, " + name);
First, we’ve enactment “Adam” successful the “name” variable. Later, we changed the worth of the aforesaid adaptable to “John.” This means that erstwhile we tally this program, the output we volition spot successful the console is “Hello, John.”
But, retrieve 1 thing:
In modern JavaScript, radical often similar utilizing “let” and “const” keywords (more connected those successful a moment) implicit “var.” Because “let” and “const” supply improved scoping rules.
let Keyword
An alternate to “var,” “let” is different keyword for creating variables successful JavaScript.
Like this:
let name = "Adam";
Now, we tin usage the adaptable “name” successful our programme to amusement the worth it stores.
For example:
let name = "Adam";
console.log("Hello, " + name);
This programme volition show "Hello, Adam" successful the console erstwhile you tally it.
If you privation to override the worth your adaptable stores, you tin bash that similar this:
var name = "Adam";
name = "Steve";
console.log("Hello, " + name);
const Keyword
“const” is akin to “let,” but declares a fixed variable.
Which means:
Once you participate a worth successful it, you can't alteration it later.
Using “const” for things similar numeric values helps forestall bugs by avoiding unintended changes aboriginal successful code.
“const” besides makes the intent clear. Other developers tin spot astatine a glimpse which variables are meant to stay unchanged.
For example:
let name = "Adam";
const age = 30;
Using “const” for "age" successful this illustration helps forestall unintentional changes to a person's age.
It besides makes it wide to different developers that "age" is meant to stay changeless passim the code.
Operators
Operators are symbols that execute operations connected variables.
Imagine you person immoderate numbers and you privation to bash mathematics with them, similar adding, subtracting, oregon comparing them.
In JavaScript, we usage peculiar symbols to bash this, and these are called operators. The main types of operators are:
Arithmetic Operators
Arithmetic operators are utilized to execute mathematical calculations connected numbers. These include:
Operator Name | Symbol | Description |
“Addition” operator | + | The “addition” relation adds numbers together |
“Subtraction” operator | - | The “subtraction” relation subtracts the right-hand worth from the left-hand value |
“Multiplication” operator | * | The “multiplication” relation multiplies numbers together |
“Division” operator | / | The “division” relation divides the left-hand fig by the right-hand number |
“Modulus” operator | % | The “modulus” relation returns a remainder aft division |
Let’s enactment each of these operators to usage and constitute a basal program:
let a = 10;
let b = 3;
let c = a + b;
console.log("c");
let d = a - b;
console.log("d");
let e = a * b;
console.log("e");
let f = a / b;
console.log("f");
let g = a % b;
console.log("g");
Here's what this programme does:
- It sets 2 variables, “a” and “b,” to 10 and 3, respectively
- Then, it uses the arithmetic operators:
- “+” to adhd the worth of “a” and “b”
- “-” to subtract the worth of “b” from “a”
- “*” to multiply the worth of “a” and “b”
- “/” to disagreement the worth of “a” by “b”
- “%” to find the remainder erstwhile “a” is divided by “b”
- It displays the results of each arithmetic cognition utilizing “console.log()”
Comparison Operators
Comparison operators comparison 2 values and instrumentality a boolean result—i.e., either existent oregon false.
They’re indispensable for penning conditional logic successful JavaScript.
The main examination operators are:
Operator Name | Symbol | Description |
“Equality” operator | == | Compares if 2 values are equal, careless of information type. For example, “5 == 5.0” would instrumentality “true” adjacent though the archetypal worth is an integer and the different is simply a floating-point fig (a numeric worth with decimal places) with the aforesaid numeric value. |
“Strict equality” operator | === | Compares if 2 values are equal, including the information type. For example, “5 === 5.0” would instrumentality “false” due to the fact that the archetypal worth is an integer and the different is simply a floating-point number, which is simply a antithetic information type. |
“Inequality” operator | != | Checks if 2 values are not equal. It doesn’t substance what benignant of values they are. For example, “5 != 10” would instrumentality “true” due to the fact that 5 does not adjacent 10. |
“Strict inequality” operator | !== | Checks if 2 values are not equal, including the information type. For example, “5 !== 5.0” would instrumentality “true” due to the fact that the archetypal worth is an integer and the different is simply a floating-point number, which is simply a antithetic information type. |
“Greater than” operator | > | Checks if the near worth is greater than the close value. For example, “10 > 5” returns “true.” |
“Less than” operator | < | Checks if the near worth is little than the close value. For example, “5 < 10” returns “true.” |
“Greater than oregon adjacent to” operator | >= | Checks if the near worth is greater than oregon adjacent to the close value. For example, “10 >= 5” returns “true.” |
“Less than oregon adjacent to” operator | <= | Checks if the near worth is little than oregon adjacent to the close value. For example, “5 <= 10” returns “true.” |
Let’s usage each these operators and constitute a basal JS programme to amended recognize however they work:
let a = 5;
let b = 5.0;
let c = 10;
if (a == b) {
console.log('true');
} else {
console.log('false');
}
if (a === b) {
console.log('true');
} else {
console.log('false');
}
if (a != c) {
console.log('true');
} else {
console.log('false');
}
if (a !== b) {
console.log('true');
} else {
console.log('false');
}
if (c > a) {
console.log('true');
} else {
console.log('false');
}
if (a < c) {
console.log('true');
} else {
console.log('false');
}
if (c >= a) {
console.log('true');
} else {
console.log('false');
}
if (a <= c) {
console.log('true');
} else {
console.log('false');
}
Here’s what this programme does:
- It sets 3 variables: “a” with a worth of 5, “b” with a worth of 5.0 (a floating-point number), and “c” with a worth of 10
- It uses the “==” relation to comparison “a” and “b.” Since “a” and “b” person the aforesaid numeric worth (5), it returns "true."
- It uses the “===” relation to comparison “a” and “b.” This time, it checks not lone the worth but besides the information type. Although the values are the same, “a” is an integer and “b” is simply a floating-point number. So, it returns "false."
- It uses the “!=” relation to comparison “a” and “c.” As “a” and “c” person antithetic values, it returns "true."
- It uses the “!==” relation to comparison “a” and “b.” Again, it considers the information type, and since “a” and “b” are of antithetic types (integer and floating-point), it returns "true."
- It uses the “>” relation to comparison “c” and “a.” Since “c” is greater than “a,” it returns "true."
- It uses the “<” relation to comparison “a” and “c.” As “a” is so little than “c,” it returns "true."
- It uses the “>=” relation to comparison “c” and “a.” Since c is greater than oregon adjacent to a, it returns "true."
- It uses the “<=” relation to comparison “a” and “c.” As “a” is little than oregon adjacent to “c,” it returns "true."
In short, this programme uses the assorted examination operators to marque decisions based connected the values of variables “a,” “b,” and “c.”
You tin spot however each relation compares these values and determines whether the conditions specified successful the if statements are met. Leading to antithetic console outputs.
Logical Operators
Logical operators let you to execute logical operations with values.
These operators are typically utilized to marque decisions successful your code, power programme flow, and make conditions for executing circumstantial blocks of code.
There are 3 main logical operators successful JavaScript:
Operator Name | Symbol | Description |
“Logical AND” operator | && | The “logical AND” relation is utilized to harvester 2 oregon much conditions. It returns “true” lone if each the conditions are true. |
“Logical OR” operator | | | | The “logical OR” relation is utilized to harvester aggregate conditions. And it returns “true” if astatine slightest 1 of the conditions is true. If each conditions are false, the effect volition beryllium “false.” |
“Logical NOT” operator | ! | The “logical NOT” relation is utilized to reverse the logical authorities of a azygous condition. If a information is true, “!” makes it “false.” And if a information is false, “!” makes it “true.” |
To amended recognize each of these operators, let’s see the examples below.
First, a “&&” (logical AND) relation example:
let age = 25;
let hasDriverLicense = true;
if (age >= 18 && hasDriverLicense) {
console.log("You can drive!");
} else {
console.log("You cannot drive.");
}
In this example, the codification checks if the property is greater than oregon adjacent to 18 and if the idiosyncratic has a driver's license. Since some conditions are true, its output is "You tin drive!"
Second, a “| |” (logical OR) relation example:
let isSunny = true;
let isWarm = true;
if (isSunny || isWarm) {
console.log("It's a great day!");
} else {
console.log("Not a great day.");
}
In this example, the codification outputs "It's a large day!" due to the fact that 1 oregon some of the conditions clasp true.
And third, a “!” (logical NOT) relation example:
let isRaining = true;
if (!isRaining) {
console.log("It's not raining. You can go outside!");
} else {
console.log("It's raining. Stay indoors.");
}
Here, the “!” relation inverts the worth of isRaining from existent to false.
So, the “if” information “!isRaining” evaluates to false. Which means the codification successful the other artifact runs, returning "It's raining. Stay indoors."
Assignment Operators:
Assignment operators are utilized to delegate values to variables. The modular duty relation is the equals motion (=). But determination are different options arsenic well.
Here’s the implicit list:
Operator Name | Symbol | Description |
“Basic assignment” operator | = | The “basic assignment” relation is utilized to delegate a worth to a variable |
“Addition assignment” operator | += | This relation adds a worth to the variable's existent worth and assigns the effect to the variable |
“Subtraction assignment” operator | -= | This relation subtracts a worth from the variable's existent worth and assigns the effect to the variable |
“Multiplication assignment” operator | *= | This relation multiplies the variable's existent worth by a specified worth and assigns the effect to the variable |
“Division assignment” operator | /= | This relation divides the variable's existent worth by a specified worth and assigns the effect to the variable |
Let’s recognize these operators with the assistance of immoderate code:
let x = 10;
x += 5;
console.log("After addition: x = ", x);
x -= 3;
console.log("After subtraction: x = ", x);
x *= 4;
console.log("After multiplication: x = ", x);
x /= 6;
console.log("After division: x = ", x);
In the codification above, we make a adaptable called “x” and acceptable it adjacent to 10. Then, we usage assorted duty operators to modify its value:
- “x += 5;” adds 5 to the existent worth of “x” and assigns the effect backmost to “x.” So, aft this operation, “x” becomes 15.
- “x -= 3;” subtracts 3 from the existent worth of “x” and assigns the effect backmost to “x.” After this operation, “x” becomes 12.
- “x *= 4;” multiplies the existent worth of “x” by 4 and assigns the effect backmost to “x.” So, “x” becomes 48.
- “x /= 6;” divides the existent worth of “x” by 6 and assigns the effect backmost to “x.” After this operation, “x” becomes 8.
At each operation, the codification prints the updated values of “x” to the console.
if-else
The “if-else” connection is simply a conditional connection that allows you to execute antithetic blocks of codification based connected a condition.
It’s utilized to marque decisions successful your codification by specifying what should hap erstwhile a peculiar information is true. And what should hap erstwhile it’s false.
Here's an illustration to exemplify however “if-else” works:
let age = 21;
if (age >= 18) {
console.log("You are an adult.");
} else {
console.log("You are a minor.");
In this example, the “age” adaptable is compared to 18 utilizing the “>=” operator.
Since “age >= 18” is true, the connection "You are an adult." is displayed. But if it weren’t, the connection "You are a minor." would’ve been displayed.
Loops
Loops are programming constructs that let you to repeatedly execute a artifact of codification arsenic agelong arsenic a specified information is met.
They’re indispensable for automating repetitive tasks.
JavaScript provides respective types of loops, including:
for Loop
A “for” loop is simply a loop that specifies "do this a circumstantial fig of times."
It's well-structured and has 3 indispensable components: initialization, condition, and increment. This makes it a almighty instrumentality for executing a artifact of codification a predetermined fig of times.
Here's the basal operation of a “for” loop:
for (initialization; condition; increment) {
// Code to be executed as long as the condition is true
}
The loop starts with the initialization (this is wherever you acceptable up the loop by giving it a starting point), past checks the condition, and executes the codification artifact if the information is true.
After each iteration, the increment is applied, and the information is checked again.
The loop ends erstwhile the information becomes false.
For example, if you privation to number from 1 to 10 utilizing a “for” loop, here’s however you would bash it:
for (let i = 1; i <= 10; i++) {
console.log(i);
}
In this example:
- The initialization portion sets up a adaptable “i” to commencement astatine 1
- The loop keeps moving arsenic agelong arsenic the information (in this case, “i <= 10”) is true
- Inside the loop, it logs the worth of “i” utilizing “console.log(i)”
- After each tally of the loop, the increment part, “i++”, adds 1 to the worth of “i”
Here's what the output volition look similar erstwhile you tally this code:
1
2
3
4
5
6
7
8
9
10
As you tin see, the “for” loop starts with “i” astatine 1. And incrementally increases it by 1 successful each iteration.
It continues until “i” reaches 10 due to the fact that the information “i <= 10” is satisfied.
The “console.log(i)” connection prints the existent worth of “i” during each iteration. And that results successful the numbers from 1 to 10 being displayed successful the console.
while Loop
A “while” loop is simply a loop that indicates "keep doing this arsenic agelong arsenic thing is true."
It's a spot antithetic from the “for” loop due to the fact that it doesn't person an initialization, condition, and increment each bundled together. Instead, you constitute the information and past enactment your codification artifact wrong the loop.
For example, if you privation to number from 1 to 10 utilizing a “while” loop, here’s however you would bash it:
let i = 1;
while (i <= 10) {
console.log(i);
i++;
}
In this example:
- You initialize “i” to 1
- The loop keeps moving arsenic agelong arsenic “i” is little than oregon adjacent to 10
- Inside the loop, it logs the worth of “i” utilizing “console.log(i)”
- “i” incrementally increases by 1 aft each tally of the loop
The output of this codification volition be:
1
2
3
4
5
6
7
8
9
10
So, with some “for” and “while” loops, you person the tools to repetition tasks and automate your code
do…while Loop
A “do...while” loop works likewise to “for” and “while” loops, but it has a antithetic syntax.
Here's an illustration of counting from 1 to 10 utilizing a “do...while” loop:
let i = 1;
do {
console.log(i);
i++;
} while (i <= 10);
In this example:
- You initialize the adaptable "i" to 1 earlier the loop starts
- The “do...while” loop begins by executing the codification block, which logs the worth of "i" utilizing “console.log(i)”
- After each tally of the loop, "i" incrementally increases by 1 utilizing “i++”
- The loop continues to tally arsenic agelong arsenic the information "i <= 10" is true
The output of this codification volition beryllium the aforesaid arsenic successful the erstwhile examples:
1
2
3
4
5
6
7
8
9
10
for…in Loop
The “for...in” loop is utilized to iterate implicit the properties of an entity (a information operation that holds key-value pairs).
It's peculiarly useful erstwhile you privation to spell done each the keys oregon properties of an entity and execute an cognition connected each of them.
Here's the basal operation of a “for...in” loop:
for (variable in object) {
// Code to be executed for each property
}
And here’s an illustration of a “for...in” loop successful action:
const person = {
name: "Alice",
age: 30,
city: "New York"
};
for (let key in person) {
console.log(key, person[key]);
}
In this example:
- You person an entity named “person” with the properties “name,” “age,” and “city”
- The “for...in” loop iterates implicit the keys (in this case, "name," "age," and "city") of the “person” object
- Inside the loop, it logs some the spot sanction (key) and its corresponding worth successful the “person” object
The output of this codification volition be:
name Alice
age 30
city New York
The “for...in” loop is simply a almighty instrumentality erstwhile you privation to execute tasks similar information extraction oregon manipulation.
Functions
A relation is simply a artifact of codification that performs a peculiar enactment successful your code. Some communal functions successful JavaScript are:
alert() Function
This relation displays a connection successful a pop-up dialog container successful the browser. It's often utilized for elemental notifications, mistake messages, oregon getting the user's attention.
Take a look astatine this illustration code:
alert("Hello, world!");
When you telephone this function, it opens a tiny pop-up dialog container successful the browser with the connection “Hello, world!” And a idiosyncratic tin admit this connection by clicking an "OK" button.
prompt() Function
This relation displays a dialog container wherever the idiosyncratic tin participate an input. The input is returned arsenic a string.
Here’s an example:
let name = prompt("Please enter your name: ");
console.log("Hello, " + name + "!");
In this code, the idiosyncratic is asked to participate their name. And the worth they supply is stored successful the sanction variable.
Later, the codification uses the sanction to greet the idiosyncratic by displaying a message, specified arsenic "Hello, [user's name]!"
confirm() Function
This relation shows a confirmation dialog container with "OK" and "Cancel" buttons. It returns “true” if the idiosyncratic clicks "OK" and “false” if they click "Cancel."
Let’s exemplify with immoderate illustration code:
const isConfirmed = confirm("Are you sure you want to continue?");
if (isConfirmed) {
console.log("true");
} else {
console.log("false");
}
When this codification is executed, the dialog container with the connection "Are you definite you privation to continue?" is displayed, and the idiosyncratic is presented with "OK" and "Cancel" buttons.
The idiosyncratic tin click either the "OK" oregon "Cancel" fastener to marque their choice.
The “confirm()” relation past returns a boolean worth (“true” oregon “false”) based connected the user's choice: “true” if they click "OK" and “false” if they click "Cancel."
console.log() Function
This relation is utilized to output messages and information to the browser's console.
Sample code:
console.log("This is the output.");
You astir apt admit it from each our codification examples from earlier successful this post.
parseInt() Function
This relation extracts and returns an integer from a string.
Sample code:
const stringNumber = "42";
const integerNumber = parseInt(stringNumber);
In this example, the “parseInt()” relation processes the drawstring and extracts the fig 42.
The extracted integer is past stored successful the adaptable “integerNumber.” Which you tin usage for assorted mathematical calculations.
parseFloat() Function
This relation extracts and returns a floating-point fig (a numeric worth with decimal places).
Sample code:
const stringNumber = "3.14";
const floatNumber = parseFloat(stringNumber);
In the illustration above, the “parseFloat()” relation processes the drawstring and extracts the floating-point fig 3.14.
The extracted floating-point fig is past stored successful the adaptable “floatNumber.”
Strings
A drawstring is simply a information benignant utilized to correspond text.
It contains a series of characters, specified arsenic letters, numbers, symbols, and whitespace. Which are typically enclosed wrong treble quotation marks (" ").
Here are immoderate examples of strings successful JavaScript:
const name = "Alice";
const number = "82859888432";
const address = "123 Main St.";
There are a batch of methods you tin usage to manipulate strings successful JS code. These are astir communal ones:
toUpperCase() Method
This method converts each characters successful a drawstring to uppercase.
Example:
let text = "Hello, World!";
let uppercase = text.toUpperCase();
console.log(uppercase);
In this example, the “toUpperCase()” method processes the substance drawstring and converts each characters to uppercase.
As a result, the full drawstring becomes uppercase.
The converted drawstring is past stored successful the adaptable uppercase, and the output successful your console is "HELLO, WORLD!"
toLowerCase() Method
This method converts each characters successful a drawstring to lowercase
Here’s an example:
let text = "Hello, World!";
let lowercase = text.toLowerCase();
console.log(lowercase);
After this codification runs, the “lowercase” adaptable volition incorporate the worth "hello, world!" Which volition past beryllium the output successful your console.
concat() Method
The “concat()” method is utilized to harvester 2 oregon much strings and make a caller drawstring that contains the merged text.
It does not modify the archetypal strings. Instead, it returns a caller drawstring that results from the operation of the archetypal strings (called the concatenation).
Here's however it works:
const string1 = "Hello, ";
const string2 = "world!";
const concatenatedString = string1.concat(string2);
console.log(concatenatedString);
In this example, we person 2 strings, “string1” and “string2.” Which we privation to concatenate.
We usage the “concat()” method connected “string1” and supply “string2” arsenic an statement (an input worth wrong the parentheses). The method combines the 2 strings and creates a caller string, stored successful the “concatenatedString” variable.
The programme past outputs the extremity effect to your console. In this case, that’s “Hello, world!”
match() Method
The “match()” method is utilized to hunt a drawstring for a specified signifier and instrumentality the matches arsenic an array (a information operation that holds a postulation of values—like matched substrings oregon patterns).
It uses a regular look for that. (A regular look is simply a series of characters that defines a hunt pattern.)
The “match()” method is highly utile for tasks similar information extraction oregon signifier validation.
Here’s a illustration codification that uses the “match()” method:
const text = "The quick brown fox jumps over the lazy dog";
const regex = /[A-Za-z]+/g;
const matches = text.match(regex);
console.log(matches);
In this example, we person a drawstring named “text.”
Then, we usage the “match()” method connected the “text” drawstring and supply a regular look arsenic an argument.
This regular expression, “/[A-Za-z]+/g,” does 2 things:
- It matches immoderate missive from “A” to “Z,” careless of whether it's uppercase oregon lowercase
- It executes a planetary hunt (indicated by “g” astatine the extremity of the regular expression). This means the hunt doesn't halt aft the archetypal lucifer is found. Instead, it continues to hunt done the full drawstring and returns each matches.
After that, each the matches are stored successful the “matches” variable.
The programme past outputs these matches to your console. In this case, it volition beryllium an array of each the words successful the condemnation "The speedy brownish fox jumps implicit the lazy dog."
charAt() Method
The “charAt()” method is utilized to retrieve the quality astatine a specified scale (position) wrong a string.
The archetypal quality is considered to beryllium astatine scale 0, the 2nd quality is astatine scale 1, and truthful on.
Here’s an example:
const text = "Hello, world!";
const character = text.charAt(7);
console.log(character);
In this example, we person the drawstring “text,” and we usage the “charAt()” method to entree the quality astatine scale 7.
The effect is the quality "w" due to the fact that "w" is astatine presumption 7 wrong the string.
replace() Method
The “replace()” method is utilized to hunt for a specified substring (a portion wrong a string) and regenerate it with different substring.
It specifies some the substring you privation to hunt for and the substring you privation to regenerate it with.
Here's however it works:
const text = "Hello, world!";
const newtext = text.replace("world", "there");
console.log(newtext);
In this example, we usage the “replace()” method to hunt for the substring "world" and regenerate it with "there."
The effect is simply a caller drawstring (“newtext”) that contains the replaced text. Meaning the output is, “Hello, there!”
substr() Method
The “substr()” method is utilized to extract a information of a string, starting from a specified scale and extending for a specified fig of characters.
It specifies the starting scale from which you privation to statesman extracting characters and the fig of characters to extract.
Here's however it works:
const text = "Hello, world!";
const substring = text.substr(7, 5);
console.log(substring);
In this example, we usage the “substr()” method to commencement extracting characters from scale 7 (which is “w”) and proceed for 5 characters.
The output is the substring "world."
(Note that the archetypal quality is ever considered to beryllium astatine scale 0. And you commencement counting from determination on.)
Events
Events are actions that hap successful the browser, specified arsenic a idiosyncratic clicking a button, a webpage finishing loading, oregon an constituent connected the leafage being hovered implicit with a mouse.
Understanding these is indispensable for creating interactive and dynamic webpages. Because they let you to respond to idiosyncratic actions and execute codification accordingly.
Here are the astir communal events supported by JavaScript:
onclick Event
The “onclick” lawsuit executes a relation oregon publication erstwhile an HTML constituent (such arsenic a fastener oregon a link) is clicked by a user.
Here’s the codification implementation for this event:
<button id="myButton" onclick="changeText()">Click me</button>
<script>
function changeText() {
let button = document.getElementById("myButton");
button.textContent = "Clicked!";
}
</script>
Now, let's recognize however this codification works:
- When the HTML leafage loads, it displays a fastener with the substance "Click me"
- When a idiosyncratic clicks connected the button, the “onclick” property specified successful the HTML tells the browser to telephone the “changeText” function
- The “changeText” relation is executed and selects the fastener constituent utilizing its id ("myButton")
- The “textContent” spot of the fastener changes to "Clicked!"
As a result, erstwhile the fastener is clicked, its substance changes from "Click me" to "Clicked!"
It's a elemental illustration of adding interactivity to a webpage utilizing JavaScript.
onmouseover Event
The “onmouseover” lawsuit occurs erstwhile a idiosyncratic moves the rodent pointer implicit an HTML element, specified arsenic an image, a button, oregon a hyperlink.
Here’s however the codification that executes this lawsuit looks:
<img id="myImage" src="image.jpg" onmouseover="showMessage()">
<script>
function showMessage() {
alert("Mouse over the image!");
}
</script>
In this example, we person an HTML representation constituent with the “id” property acceptable to "myImage."
It besides has an “onmouseover” property specified, which indicates that erstwhile the idiosyncratic hovers the rodent pointer implicit the image, the "showMessage ()" relation should beryllium executed.
This relation displays an alert dialog with the connection "Mouse implicit the image!"
The “onmouseover” lawsuit is utile for adding interactivity to your web pages. Such arsenic providing tooltips, changing the quality of elements, oregon triggering actions erstwhile the rodent moves implicit circumstantial areas of the page.
onkeyup Event
The “onkeyup” is an lawsuit that occurs erstwhile a idiosyncratic releases a cardinal connected their keyboard aft pressing it.
Here’s the codification implementation for this event:
<input type="text" id="myInput" onkeyup="handleKeyUp()">
<script>
function handleKeyUp() {
let input = document.getElementById("myInput");
let userInput = input.value;
console.log("User input: " + userInput);
}
</script>
In this example, we person an HTML substance input constituent <input> with the “id” property acceptable to "myInput."
It besides has an “onkeyup” property specified, indicating that erstwhile a cardinal is released wrong the input field, the "handleKeyUp()" relation should beryllium executed.
Then, erstwhile the idiosyncratic types oregon releases a cardinal successful the input field, the “handleKeyUp()” relation is called.
The relation retrieves the input worth from the substance tract and logs it to the console.
This lawsuit is commonly utilized successful forms and substance input fields to respond to a user’s input successful existent time.
It’s adjuvant for tasks similar auto-suggestions and quality counting, arsenic it allows you to seizure users’ keyboard inputs arsenic they type.
onmouseout Event
The “onmouseout” lawsuit occurs erstwhile a idiosyncratic moves the rodent pointer retired of the country occupied by an HTML constituent similar an image, a button, oregon a hyperlink.
When the lawsuit is triggered, a predefined relation oregon publication is executed.
Here’s an example:
<img id="myImage" src="image.jpg" onmouseout="hideMessage()">
<script>
function hideMessage() {
alert("Mouse left the image area!");
}
</script>
In this example, we person an HTML representation constituent with the “id” property acceptable to "myImage."
It besides has an “onmouseout” property specified, indicating that erstwhile the idiosyncratic moves the rodent cursor retired of the representation area, the "hideMessage()" relation should beryllium executed.
Then, erstwhile the idiosyncratic moves the rodent cursor retired of the representation area, a JavaScript relation called “hideMessage()” is called.
The relation displays an alert dialog with the connection "Mouse near the representation area!"
onload Event
The “onload” lawsuit executes a relation oregon publication erstwhile a webpage oregon a circumstantial constituent wrong the leafage (such arsenic an representation oregon a frame) has finished loading.
Here’s the codification implementation for this event:
<body onload="initializePage()">
<script>
function initializePage() {
alert("Page has finished loading!");
}
</script>
In this example, erstwhile the webpage has afloat loaded, the “initializePage()” relation is executed, and an alert with the connection "Page has finished loading!" is displayed.
onfocus Event
The “onfocus” lawsuit triggers erstwhile an HTML constituent similar an input tract receives absorption oregon becomes the progressive constituent of a user’s input oregon interaction.
Take a look astatine this illustration code:
<input type="text" id="myInput" onfocus="handleFocus()">
<script>
function handleFocus() {
alert("Input field has received focus!");
}
</script>
In this example, we person an HTML substance input constituent <input> with the “id” property acceptable to "myInput."
It besides has an “onfocus” property specified. Which indicates that erstwhile the idiosyncratic clicks connected the input tract oregon tabs into it, the "handleFocus()" relation volition beryllium executed.
This relation displays an alert with the connection "Input tract has received focus!"
The “onfocus” lawsuit is commonly utilized successful web forms to supply ocular cues (like changing the inheritance colour oregon displaying further information) to users erstwhile they interact with input fields.
onsubmit Event
The “onsubmit” lawsuit triggers erstwhile a idiosyncratic submits an HTML form. Typically by clicking a "Submit" fastener oregon pressing the "Enter" cardinal wrong a signifier field.
It allows you to specify a relation oregon publication that should beryllium executed erstwhile the idiosyncratic attempts to taxable the form.
Here’s a codification sample:
<form id="myForm" onsubmit="handleSubmit()">
<input type="text" name="username" placeholder="Username">
<input type="password" name="password" placeholder="Password">
<button type="submit">Submit</button>
</form>
<script>
function handleSubmit() {
let form = document.getElementById("myForm");
alert("Form submitted!");
}
</script>
In this example, we person an HTML signifier constituent with the “id” property acceptable to "myForm."
It besides has an “onsubmit” property specified, which triggers the "handleSubmit()" relation erstwhile a idiosyncratic submits the form.
This relation shows an alert with the connection "Form submitted!"
Numbers and Math
JavaScript supports tons of methods (pre-defined functions) to woody with numbers and bash mathematical calculations.
Some of the methods it supports include:
Math.abs() Method
This method returns the implicit worth of a number, ensuring the effect is positive.
Here's an illustration that demonstrates the usage of the “Math.abs()” method:
let negativeNumber = -5;
let positiveNumber = Math.abs(negativeNumber);
console.log("Absolute value of -5 is: " + positiveNumber);
In this code, we commencement with a antagonistic fig (-5). By applying “Math.abs(),” we get the implicit (positive) worth of 5.
This method is adjuvant for scenarios wherever you request to guarantee that a worth is non-negative, careless of its archetypal sign.
Math.round() Method
This method rounds a fig up to the nearest integer.
Sample code:
let decimalNumber = 3.61;
let roundedUpNumber = Math.round(decimalNumber);
console.log("Ceiling of 3.61 is: " + roundedUpNumber);
In this code, we person a decimal fig (3.61). Applying “Math.round()” rounds it up to the nearest integer. Which is 4.
This method is commonly utilized successful scenarios erstwhile you privation to circular up quantities, specified arsenic erstwhile calculating the fig of items needed for a peculiar task oregon erstwhile dealing with quantities that can't beryllium fractional.
Math.max() Method
This method returns the largest worth among the provided numbers oregon values. You tin walk aggregate arguments to find the maximum value.
Here's an illustration that demonstrates the usage of the “Math.max()” method:
let maxValue = Math.max(5, 8, 12, 7, 20, -3);
console.log("The maximum value is: " + maxValue);
In this code, we walk respective numbers arsenic arguments to the “Math.max()” method.
The method past returns the largest worth from the provided acceptable of numbers, which is 20 successful this case.
This method is commonly utilized successful scenarios similar uncovering the highest people successful a crippled oregon the maximum somesthesia successful a acceptable of information points.
Math.min() Method
The “Math.min()” method returns the smallest worth among the provided numbers oregon values.
Sample code:
let minValue = Math.min(5, 8, 12, 7, 20, -3);
console.log("The minimum value is: " + minValue);
In this code, we walk respective numbers arsenic arguments to the “Math.min()” method.
The method past returns the smallest worth from the fixed acceptable of numbers, which is -3.
This method is commonly utilized successful situations similar identifying the shortest region betwixt aggregate points connected a representation oregon uncovering the lowest somesthesia successful a acceptable of information points.
Math.random() Method
This method generates a random floating-point fig betwixt 0 (inclusive) and 1 (exclusive).
Here’s immoderate illustration code:
const randomValue = Math.random();
console.log("Random value between 0 and 1: " + randomValue);
In this code, we telephone the “Math.random()” method, which returns a random worth betwixt 0 (inclusive) and 1 (exclusive).
It's often utilized successful applications wherever randomness is required.
Math.pow() Method
This method calculates the worth of a basal raised to the powerfulness of an exponent.
Let’s look astatine an example:
let base = 2;
let exponent = 3;
let result = Math.pow(base, exponent);
console.log(`${base}^${exponent} is equal to: ${result}`)
In this code, we person a basal worth of 2 and an exponent worth of 3. By applying “Math.pow(),” we cipher 2 raised to the powerfulness of 3, which is 8.
Math.sqrt() Method
This method computes the quadrate basal of a number.
Take a look astatine this illustration code:
let number = 16;
const squareRoot = Math.sqrt(number);
console.log(`The square root of ${number} is: ${squareRoot}`);
In this code, we person the fig 16. By applying “Math.sqrt(),” we cipher the quadrate basal of 16. Which is 4.
Number.isInteger() Method
This method checks whether a fixed worth is an integer. It returns existent if the worth is an integer and mendacious if not.
Here's an illustration that demonstrates the usage of the “Number.isInteger()” method:
let value1 = 42;
let value2 = 3.14;
let isInteger1 = Number.isInteger(value1);
let isInteger2 = Number.isInteger(value2);
console.log(`Is ${value1} an integer? ${isInteger1}`);
console.log(`Is ${value2} an integer? ${isInteger2}`);
In this code, we person 2 values, “value1” and “value2.” We usage the “Number.isInteger()” method to cheque whether each worth is an integer:
- For “value1” (42), “Number.isInteger()” returns “true” due to the fact that it's an integer
- For “value2” (3.14), “Number.isInteger()” returns “false” due to the fact that it's not an integer—it contains a fraction
Date Objects
Date objects are utilized to enactment with dates and times.
They let you to create, manipulate, and format day and clip values successful your JavaScript code.
Some communal methods include:
getDate() Method
This method retrieves the existent time of the month. The time is returned arsenic an integer, ranging from 1 to 31.
Here's however you tin usage the “getDate()” method:
let currentDate = new Date();
let dayOfMonth = currentDate.getDate();
console.log(`Day of the month: ${dayOfMonth}`);
In this example, “currentDate” is simply a “date” entity representing the existent day and time.
We past usage the “getDate()” method to retrieve the time of the period and store it successful the “dayOfMonth” variable.
Finally, we show the time of the period utilizing “console.log().”
getDay() Method
This method retrieves the existent time of the week.
The time is returned arsenic an integer, with Sunday being 0, Monday being 1, and truthful on. Up to Saturday being 6.
Sample code:
let currentDate = new Date();
let dayOfWeek = currentDate.getDay();
console.log(`Day of the week: ${dayOfWeek}`);
Here, “currentDate” is simply a day entity representing the existent day and time.
We past usage the “getDay()” method to retrieve the time of the week and store it successful the “dayOfWeek” variable.
Finally, we show the time of the week utilizing “console.log().”
getMinutes()Method
This method retrieves the minutes information from the contiguous day and time.
The minutes volition beryllium an integer value, ranging from 0 to 59.
Sample code:
let currentDate = new Date();
let minutes = currentDate.getMinutes();
console.log(`Minutes: ${minutes}`);
In this example, “currentDate” is simply a “date” entity representing the existent day and time.
We usage the “getMinutes()” method to retrieve the minutes constituent and store it successful the minutes variable.
Finally, we show the minutes utilizing “console.log().”
getFullYear() Method
This method retrieves the existent year. It’ll beryllium a four-digit integer.
Here’s immoderate illustration code:
let currentDate = new Date();
let year = currentDate.getFullYear();
console.log(`Year: ${year}`);
Here, “currentDate” is simply a day entity representing the existent day and time.
We usage the “getFullYear()” method to retrieve the twelvemonth and store it successful the twelvemonth variable.
We past usage “console.log()” to show the year.
setDate() Method
This method sets the time of the month. By changing the time of the period worth wrong the “date” object.
Sample code:
let currentDate = new Date();
currentDate.setDate(15);
console.log(`Updated date: ${currentDate}`);
In this example, “currentDate” is simply a “date” entity representing the existent day and time.
We usage the “setDate()” method to acceptable the time of the period to 15. And the “date” entity is updated accordingly.
Lastly, we show the updated day utilizing “console.log().”
How to Identify JavaScript Issues
JavaScript errors are common. And you should code them arsenic soon arsenic you can.
Even if your codification is error-free, hunt engines whitethorn person occupation rendering your website contented correctly. Which tin forestall them from indexing your website properly.
As a result, your website whitethorn get little postulation and visibility.
You tin cheque to spot if JS is causing immoderate rendering issues by auditing your website with Semrush’s Site Audit tool.
Open the instrumentality and participate your website URL. Then, click “Start Audit.”
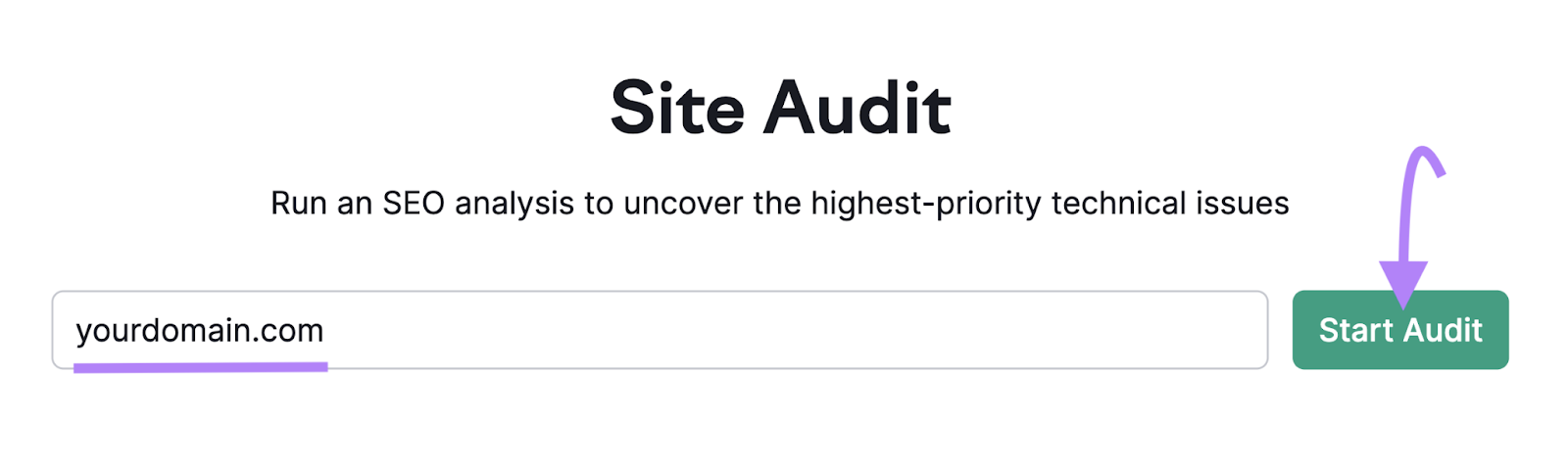
A caller model volition popular up. Here, acceptable the scope of your audit.
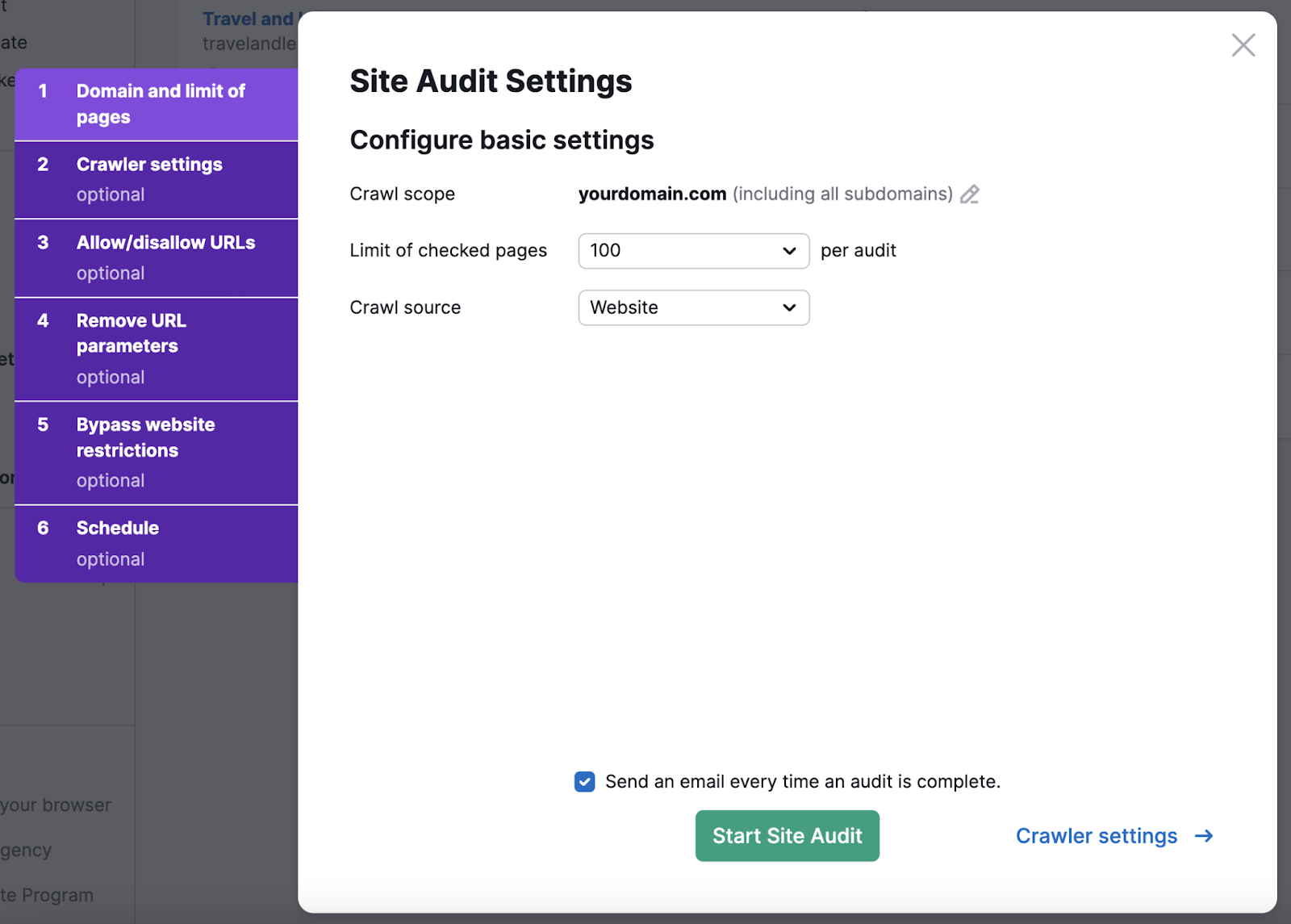
After that, spell to “Crawler settings” and alteration the “JS rendering” option. Then, click “Start Site Audit.”
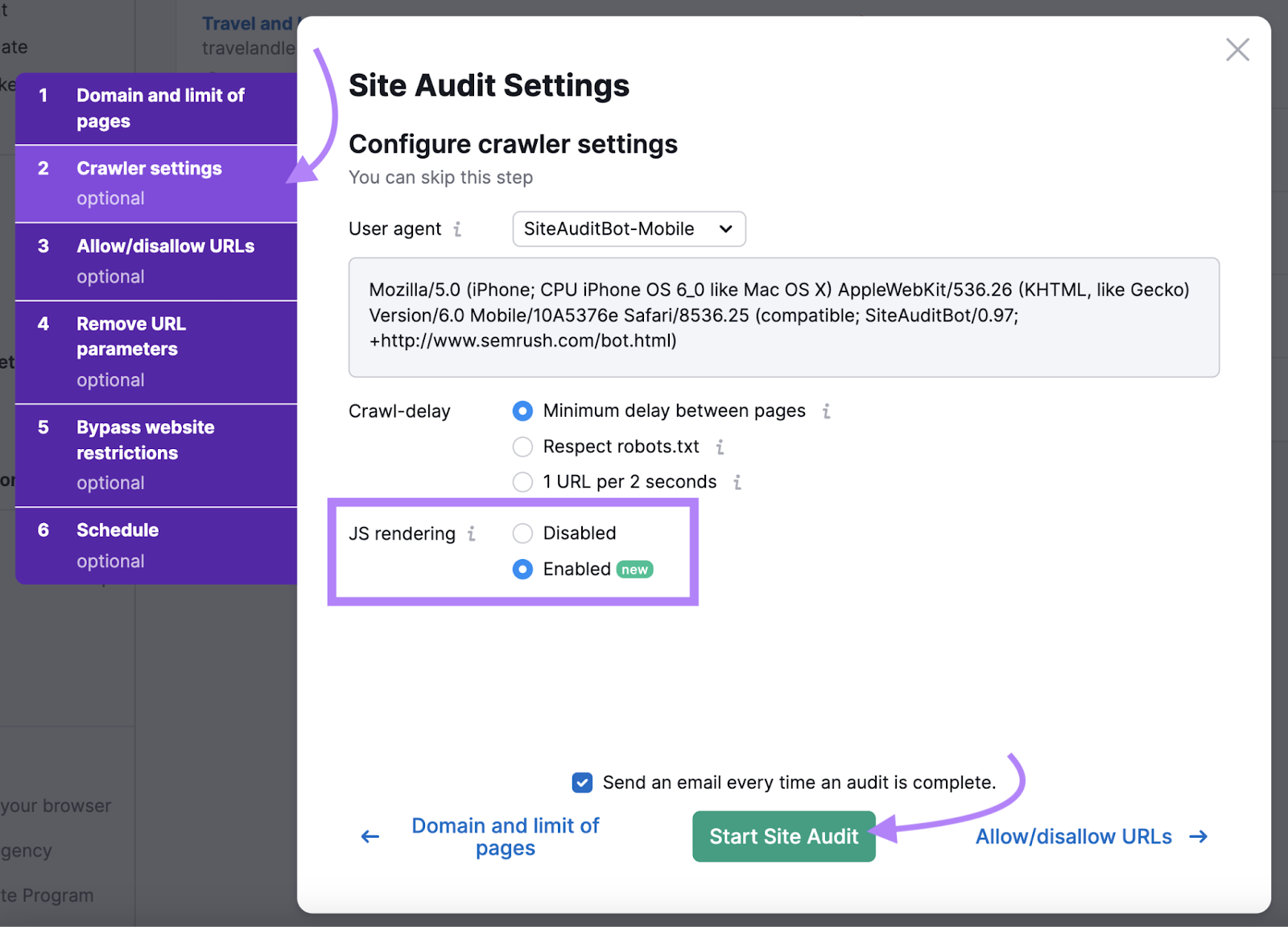
The instrumentality volition commencement auditing your site. After the audit is complete, navigate to the “JS Impact” tab.
You’ll spot whether definite elements (links, content, title, etc.) are rendered otherwise by the browser and the crawler.
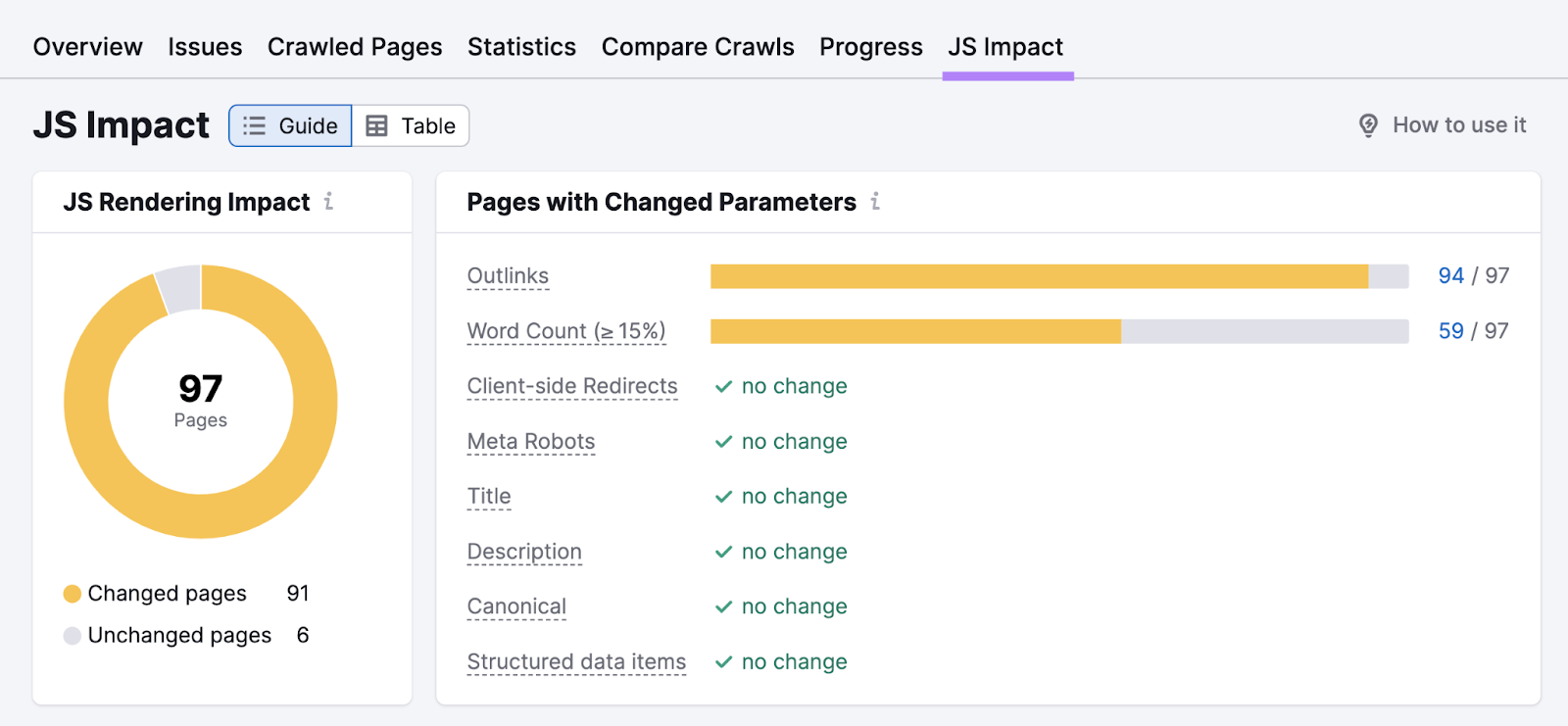
For your website to beryllium optimized, you should purpose to minimize the differences betwixt the browser and the crawler versions of your webpages.
This volition guarantee that your website contented is afloat accessible and indexable by hunt engines .
To minimize the differences, you should travel the champion practices for JavaScript SEO and instrumentality server-side rendering (SSR).
Even Google recommends doing this.
Why?
Because SSR minimizes the disparities betwixt the mentation of your webpages that browser and hunt engines see.
You tin past rerun the audit to corroborate that the issues are resolved.
Get Started with JavaScript
Learning however to codification successful JavaScript is simply a skill. It’ll instrumentality clip to maestro it.
Thankfully, we’ve enactment unneurotic a useful cheat expanse to assistance you larn the basics of JavaScript and get started with your coding journey.
You tin download the cheat sheet arsenic a PDF record oregon presumption it online.
We anticipation this cheat expanse volition assistance you get acquainted with the JavaScript language. And boost your assurance and skills arsenic a coder.